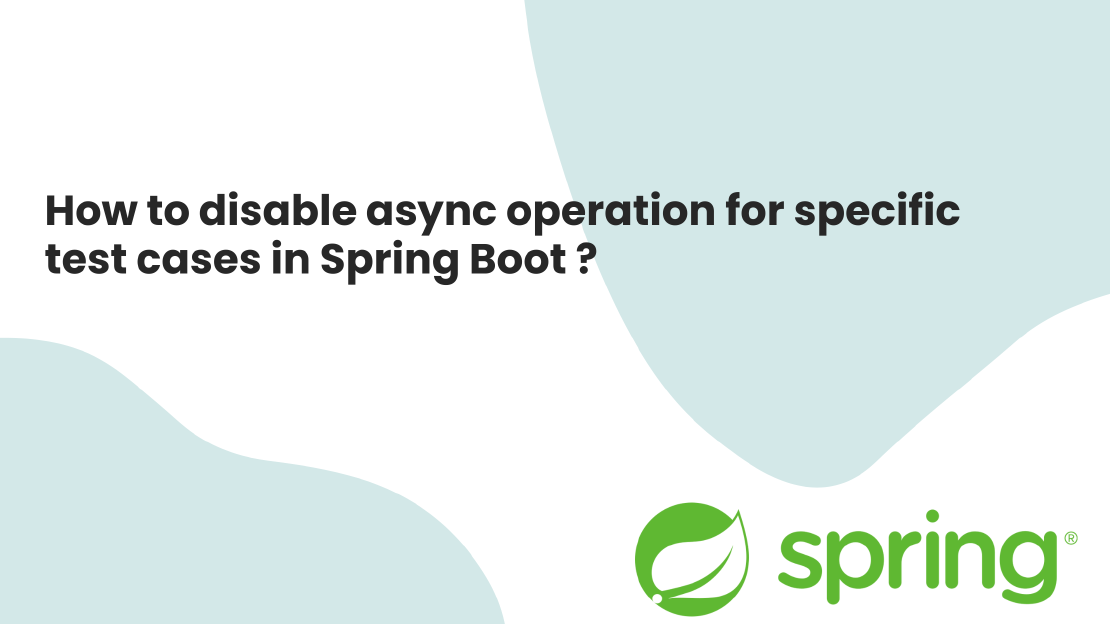
How to disable async operation for specific test cases in Spring Boot
I was facing a challenge with running test cases that include async methods in my Spring Boot application. The test cases failed because the async …
@RequestHeader
annotation is used to bind a request header to a method argument in a controller. In other words, it allows us to read http headers in the controllers
For instance let’s say your request has the following headers:
Accept-Language tr,en-gb;q=0.7,en;q=0.3
Accept-Encoding gzip
If you want to get the headers value of Accept-Encoding
and Accept-Language
:
import org.springframework.web.bind.annotation.RequestHeader;
@RestController
public class ApiController {
@GetMapping
public ResponseEntity<...> handleRequest(
@RequestHeader(name = "Accept-Encoding") String encoding,
@RequestHeader(name = "Accept-Language") String acceptLanguage) {
// ...
}
}
@RequestHeader(name = "Accept-Encoding") String encoding
: Get the value of header called Accept-Encoding@RequestHeader(name = "Accept-Language") String acceptLanguage
: Get the value of header called Accept-LanguageIf header value contains multiple values such as Accept-Language, you can use following types:
Map<String, String>
MultiValueMap<String, String>
HttpHeaders
List<String>
import org.springframework.web.bind.annotation.RequestHeader;
import org.springframework.http.HttpHeaders;
@RestController
public class ApiController {
@GetMapping
public ResponseEntity<...> handleRequest(
@RequestHeader(name = "Accept-Encoding") String encoding,
@RequestHeader(name = "Accept-Language") HttpHeaders headers) {
// ...
}
}
I was facing a challenge with running test cases that include async methods in my Spring Boot application. The test cases failed because the async …
There are 3 ways to change default running port in spring boot application: By default, the embedded server starts on port 8080. 1- Use Property files …