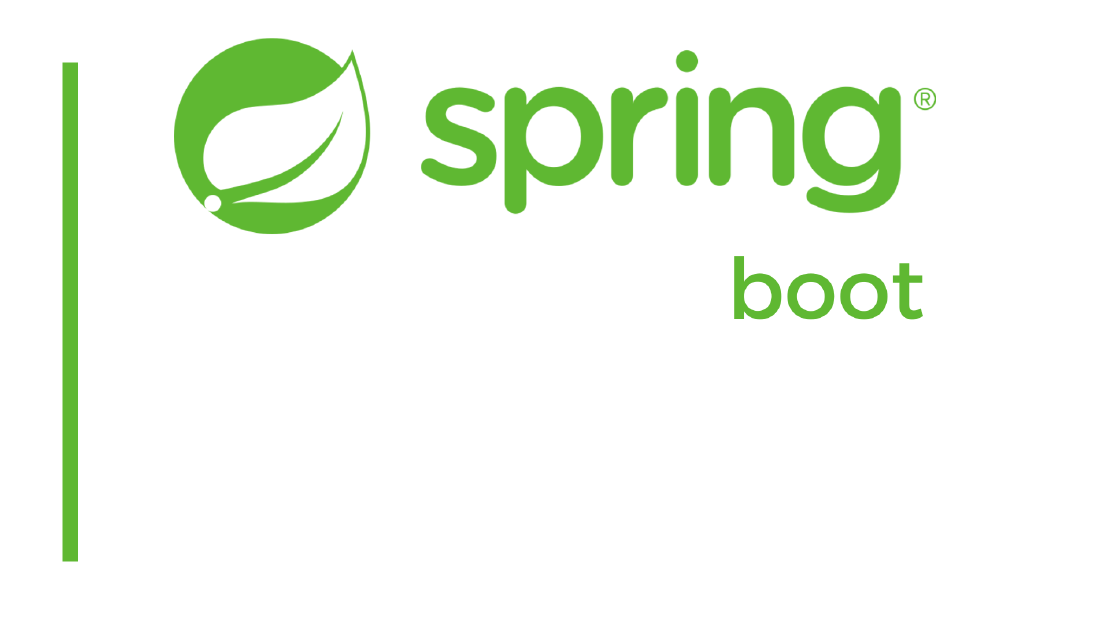
How to change running port in spring boot?
There are 3 ways to change default running port in spring boot application: By default, the embedded server starts on port 8080. 1- Use Property files …
I was facing a challenge with running test cases that include async methods in my Spring Boot application. The test cases failed because the async method didn’t complete before the test case finished. I have discovered that when you remove or disable the async configuration, the test cases pass.
To specifically disable async operation for certain test cases, we can use the SyncTaskExecutor class provided by Spring, which executes tasks synchronously in the calling thread. Here’s how you can set it up:
public class DisableAsyncConfiguration {
@Bean
public Executor taskExecutor() {
return new SyncTaskExecutor();
}
}
SyncTaskExecutor
is a class which is TaskExecutor implementation that executes each task synchronously in the calling thread.
@Import
annotation. For example:// import the configuration, from now on async operation will run on SyncTaskExecutor for these test class
@Import(value = {DisableAsyncConfiguration.class})
public class AnyTest {
@Test
void testIncludesAsyncCall() throws Exception {
// ...
}
}
By importing the DisableAsyncConfiguration class, the async operations in your test class will be executed synchronously using the SyncTaskExecutor, allowing you to properly test them.
There are 3 ways to change default running port in spring boot application: By default, the embedded server starts on port 8080. 1- Use Property files …
It is so easy to learn your spring boot version. To learn that you have to look at the following files (according to project tool you are using): If …