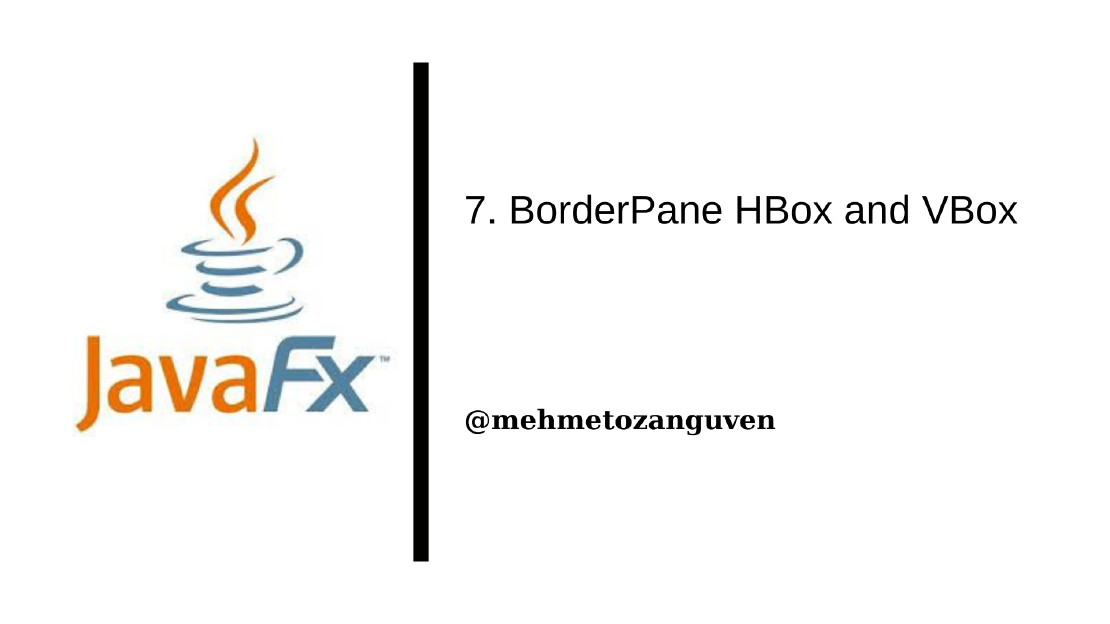
JavaFX BorderPane HBox and VBox
BorderPane BorderPane can be useful for displaying one large central component with four smaller components at left, top, right and bottoms Note you …
grid.setHGap(size);
gris.setVGap(size);
grid.add(child, column, row);
grid.add(child, column, row, columnCount, rowCount);
gridpane.getRowConstraints().addAll(
new RowConstraints(100), // row 0 has height 100 pixels
new RowConstraints(150), // row 1 has height 150 pixels
new RowConstraints(100), // row 2 has height 100 pixels
new RowConstraints(200), // row 3 has height 200 pixeds
);
For example, to force a five-column gridpane to fill the available width and to force all columns to have the same size:
for (int i = 0; i < 5; i++) {
ColumnConstraints constraints = new ColumnConstraints();
constraints.setPercentWidth(20);
gridpane.getColumnConstraints().add(constraints);
}
If we want each (row, column) pair to take equal size, then we can use TilePane. We can set the number of columns and rows by calling: tpane.setPrefColumns(cols); & tpane.setPrefRows(rows);
For instance if we set column to 1 then we actually get a VBox, and if we set row to 1 then we actually get an HBox.
Some constructors in the TilePane:
public TilePane() {
super();
}
public TilePane(double hgap, double vgap) {
super();
setHgap(hgap);
setVgap(vgap);
}
public TilePane(Node...children) {
super();
getChildren().addAll(children);
}
BorderPane BorderPane can be useful for displaying one large central component with four smaller components at left, top, right and bottoms Note you …
In this blog post, let’s talk about laying out the components in the JavaFX. We need a pre-defined layout solution because computing the …