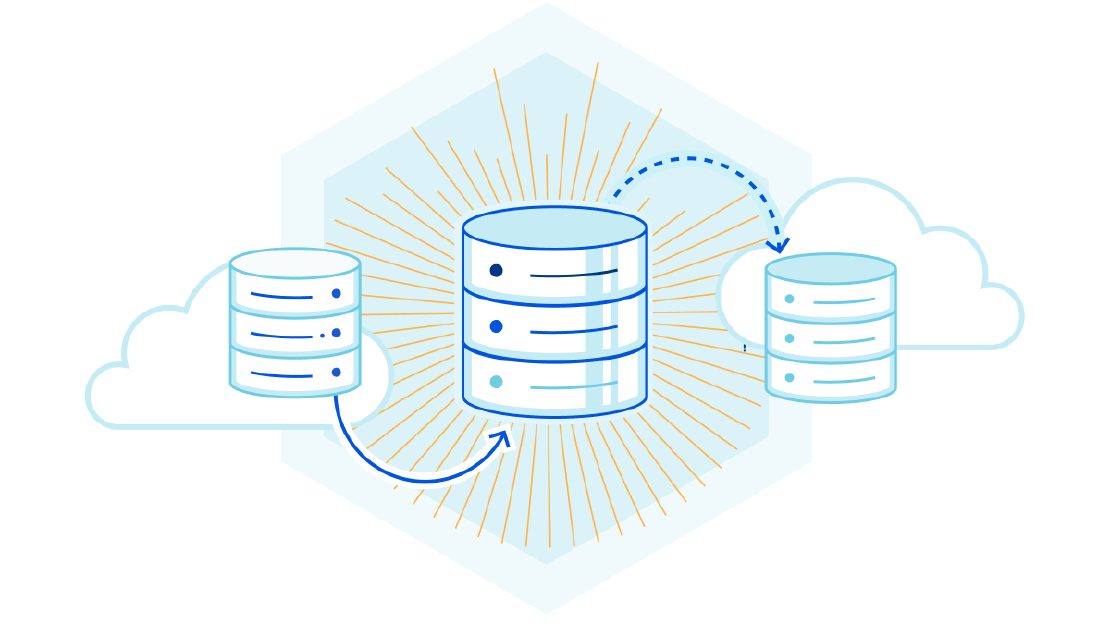
Creating entity with includes nested data structures in Hibernate 6 (and in Spring Boot 3)
Sometimes your entity might need store nested data structures such as Map<String, Map<String, String> or list of list etc.. If you want to …
Let’s assume that you have the following message template in which clients send the text with the correct parameter. Here is the template:
Hello,
Reminds you that you have an appointment on {{1}}. We wish you a nice day
{{2}}
Now, suppose you receive a sample request from a client (sent to our backend API) that looks like this:
Hello,
Reminds you that you have an appointment on 2023-05-13 10:00. We wish you a nice day
CompanyName
And our goal is to verify whether the client’s request matches the defined template. This can be achieved using Java’s Pattern class for pattern matching.
public class TemplateVerification {
public static void main(String[] args) {
String template = """
Hello,
Reminds you that you have an appointment on {{1}}. We wish you a nice day
{{2}}
""";
String requestFromClient = """
Hello,
Reminds you that you have an appointment on 2023-05-13 10:00. We wish you a nice day
CompanyName
""";
// create a new String variable named trimmedTemplate
// and assigns it the value of template after removing any leading or trailing whitespace.
String trimmedTemplate = template.strip();
// Create a regular expression pattern
// by replacing the placeholders {{1}} and {{2}} in the trimmedTemplate string with the regular expression (.*), which matches any sequence of characters.
String regex = trimmedTemplate.replace("{{1}}", "(.*)").replace("{{2}}", "(.*)");
// Compile the regular expression pattern stored in the regex variable into a Pattern object.
// The Pattern.DOTALL flag is used to enable the dot character (.) to match all characters, including line terminators.
Pattern pattern = Pattern.compile(regex, Pattern.DOTALL);
// Create a matcher for the incoming text
Matcher matcher = pattern.matcher(requestFromClient.strip());
if (matcher.matches()) {
System.out.println("Text matches the template.");
} else {
System.out.println("Text does not match the template.");
}
}
}
Let’s discuss why we replace the template variables ({{1}} and {{2}}
) with (.*)
:
The
(.*)
in regex is a common pattern that matches any sequence of characters. The dot(.)
matches any character except line terminators, and the asterisk(*)
indicates that the preceding pattern(.)
can occur zero or more times.
{{1}}
and {{2}}
in string with (.*)
, we create a regex pattern that will match any content in place of those placeholders. The (.*)
essentially acts as a wildcard, allowing any text to be matched at those positions.{{1}}
and “CompanyName” at the position of {{2}}
, the regex pattern (.*?)
will match “2023-05-13 10:00” at the position of {{1}}
and “CompanyName” at the position of {{2}}
In summary, by replacing {{1}}
and {{2}}
with (.*)
, I create a flexible regex pattern that can match any content at those respective positions in the requestFromClient string.
We can enhance validation code for instance by restriction max allowed lenght for variables.
public class TemplateVerificationEnhanced {
private static final int MAX_VARIABLE_SIZE = 20; // Maximum allowed size for template variables
public static void main(String[] args) {
String template = """
Hello,
Reminds you that you have an appointment on {{1}}. We wish you a nice day
{{2}}
""";
String requestFromClient = """
Hello,
Reminds you that you have an appointment on 2023-05-13 10:00. We wish you a nice day
CompanyName
""";
// Remove leading spaces and newlines from the template
String trimmedTemplate = template.strip();
// Replace all variables {{1}}, {{2}}, ... {{n}} in the template with regular expressions
String regex = trimmedTemplate.replaceAll("\\{\\{\\d+\\}\\}", "(.{1," + MAX_VARIABLE_SIZE + "})");
Pattern pattern = Pattern.compile(regex, Pattern.DOTALL);
Matcher matcher = pattern.matcher(requestFromClient.strip());
if (matcher.matches()) {
System.out.println("Text matches the template.");
} else {
System.out.println("Text does not match the template.");
}
}
}
Here is the basic explanation for the replaceAll
line:
{{1}}, {{2}}, ..., {{n}}
in the trimmedTemplate string with a regular expression pattern.\\{\\{\\d+\\}\\}
matches the exact format of {{n}}
where n represents a positive integer.(.{1,20})
matches any character sequence with a minimum length of 1 and a maximum length of MAX_VARIABLE_SIZE(20)By using these two methods you can be sure that everything is matches with your defined templates, texts etc …
Sometimes your entity might need store nested data structures such as Map<String, Map<String, String> or list of list etc.. If you want to …
In this tutorial, we will integrate Spring MVC with gulp and webpack. As you know creating Spring MVC project with Thymelaef project is so easy. But …