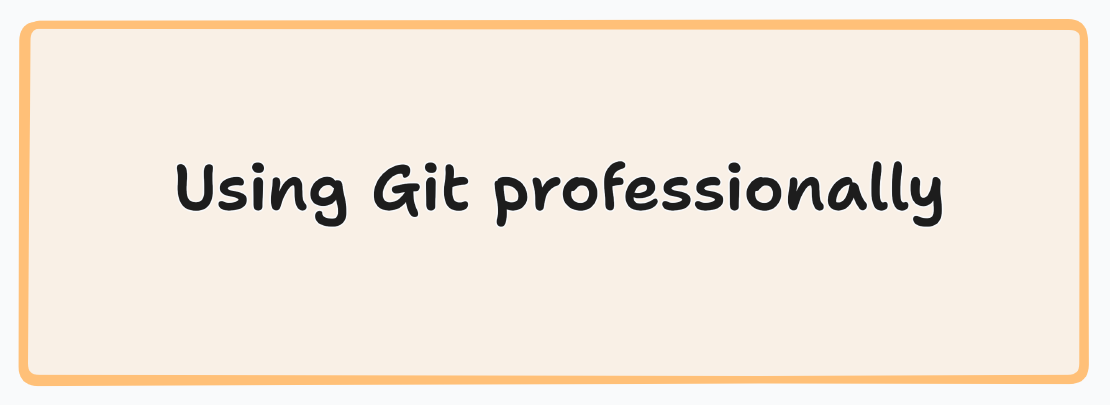
Using Git Professionally
In this long blog, we are going to improve our git knowledge. I will try to explain the some concepts behing the basic things in git. Writing perfect …
Git is a tool for keeping track of changes in computer programs. It’s free to use and lots of people in the software field use it. Now, let’s check out some questions that might come up in an interview.
git init
and git clone
?Both of them are used to create git repository.
git clone
: clones a repository into a newly created directory. Mainly used to download after developers repositories'.git init
: creates an empty git repository. It is a directory in which contains .git
directory.Three Trees of git actually refers to the Git’s internal state management system. These trees are node and pointer based data structures in which Git uses to tract a timeline of edits.
git add
command.
git ls-files
git ls-files
list all files in which git has aware of.git commit
command, it takes the changes you made and puts them into a permanent snapshot that stays in the Commit History.
git status
commandgit add
and git commit
commandsgit add
: Adds new or changed files in our working directory to the Git staging areagit commit
: It creates a permanent copy of the currently staged changes.git checkout
command-b
=> git checkout -b myNewBranch
-b
, then it switches you to specified branchUse git checkout
:
# switch to master branch
git checkout master
# switch to branch called dummyBranch if exists
git checkout dummyBranch
There are some situations for this question:
git checkout
command. Example => git checkout fileName
Let’s say you made a mistake in your your last commit but not pushed to remote repository.
git reset --soft HEAD~
Let’s say you made local commits but not pushed to remote repository yet. And you want to change your commit or reset things back to previous good commit.
git status
), you have to find your unique hash such as 2cf1254ga…git reset
commit, you may search on the internet)
git reset 2cf1254ga
or if you want git reset --hard 2cf1254ga
The safest option is the git reset (without –hard) , in this case, commits will be removed, but the changes will appear as uncommitted, giving you access to the code.
Let’s say you made a changes and pushed to the remote repository, but you want to revert it.
git revert 2cf1254ga
or git rever 2cf1254ga --no-edit
--no-edit
, git expects a revert commit message from you. You can bypass the revert-commit message (git will automatically add default message)git push
Use git log
command:
$ git log
commit 21815196d4f85aa81a1aec93a112540827905e40 (HEAD -> master, origin/master)
Author: mehmetozanguven ...
Date: Tue Sep ...
Add new blog for git
...
git show
.gitignore
.gitignore
specifices a patterngit merge
commandLet’s say you want to add your implementation in the branch called newFeature
into your master branch. Then you may run the following commands:
# switch to master
git checkout master
# merge branch into master
git merge newFeature
Let’s also talk about some key points:
git fetch & git pull
git fetch
: retrieves changes from the remote repository without automatically merging them into local branch.git pull
: It does both fetching and merging a single step.git remote
commandgit remote -v
: list the remote connections:$ git remote -v
origin [email protected]:mehmetozanguven/mehmetozanguven.com.git (fetch)
origin [email protected]:mehmetozanguven/mehmetozanguven.com.git (push)
git remote add <name> <url>
: creates a new connection to a remote repository. You will be use <name>
as a shortcut for the <url>
in other Git commandsgit remote rename <oldName> <newName>
: rename a remote connectiongit remote rm <name>
: remove the connection to the remote repositoryIn this long blog, we are going to improve our git knowledge. I will try to explain the some concepts behing the basic things in git. Writing perfect …
It is crucial to capture long running method execution in your application (whether it is an API or traditional mvc application). You should also …