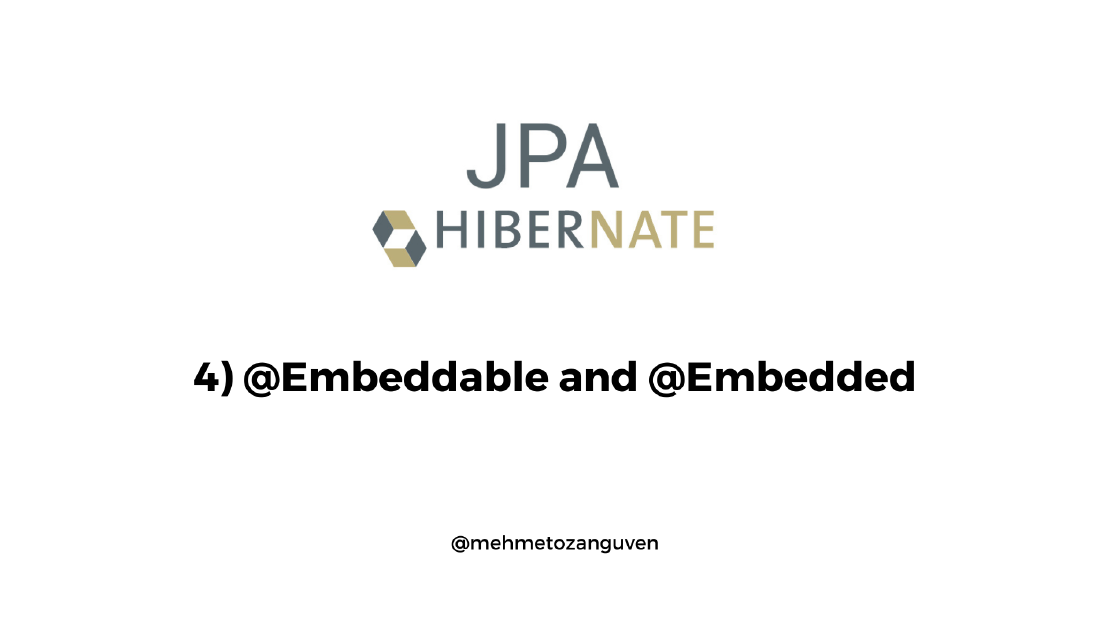
JPA Fundamentals & Hibernate - 4) @Embeddable and @Embedded
In this post, we are going to learn when and how to use embaddable object in Java and JPA. Github Link If you only need to see the code, here is the …
In this article, we are going to find out how to create composite keys in JPA.
Composite key is the key which composed of multiple columns.
If you only need to see the code, here is the github link
Let’s say we have table called departmant
and it has composed primary keys (for column code and number).
Run the following sql query:
CREATE TABLE department
(
code VARCHAR(100),
number INT,
name VARCHAR(100),
PRIMARY KEY(code, number)
);
We have options to create composite key:
@IdClass
@Id
Serializable
interface (JPA specification)@IdClass(YourComposedClass.class)
@Id
@Entity
@Table(name = "department")
public class Department {
@Id
private String code;
@Id
private int number;
private String name;
// getters and setters..
}
Fields in the composed key class must match with the fields annotated with @Id
in the entity class
public class DepartmentPK implements Serializable {
private String code;
private int number;
// getters and setters
}
@IdClass
@IdClass(DepartmentPK.class)
public class Department {}
Let’s create an department entity and persist it:
public class Main {
public static void main(String[] args) {
Department department = new Department();
department.setNumber(10);
department.setCode("AB");
department.setName("test");
try {
entityManager.getTransaction().begin();
entityManager.persist(department);
entityManager.getTransaction().commit();
}catch (Exception e) {
System.out.println("Exception: " + e.getMessage());
} finally {
entityManager.close();
}
}
}
Then run the select query:
select * from department ;
code | number | name
------+--------+------
AB | 10 | test
(1 row)
Almost the same procedure as the @Embeddable /@Embedded
in the previous blog. Only difference is that we should use @EmbeddedId
and embeddable object must implements Serializable interface
department_two
CREATE TABLE department_two
(
code VARCHAR(100),
number INT,
name VARCHAR(100),
PRIMARY KEY(code, number)
);
@Embeddable
public class DepartmentEmbeddablePK implements Serializable {
private int number;
private String code;
// getters and setters
}
@Entity
@Table(name = "department_two")
public class DepartmentTwo {
@EmbeddedId
@AttributeOverrides({
@AttributeOverride(name = "number", column = @Column(name = "number")),
@AttributeOverride(name = "code", column = @Column(name = "code")),
})
private DepartmentEmbeddablePK departmentEmbeddablePK;
private String name;
// getters and setters
}
public class Main {
public static void main(String[] args) {
DepartmentEmbeddablePK departmentEmbeddablePK = new DepartmentEmbeddablePK();
departmentEmbeddablePK.setNumber(11);
departmentEmbeddablePK.setCode("BC");
DepartmentTwo departmentTwo = new DepartmentTwo();
departmentTwo.setName("test2");
departmentTwo.setDepartmentEmbeddablePK(departmentEmbeddablePK);
try {
entityManager.getTransaction().begin();
entityManager.persist(departmentTwo);
entityManager.getTransaction().commit();
}catch (Exception e) {
System.out.println("Exception: " + e.getMessage());
} finally {
entityManager.close();
}
}
}
In this post, we are going to learn when and how to use embaddable object in Java and JPA. Github Link If you only need to see the code, here is the …
In this post, we are going to learn @Enumarated and @Temporal annotations, how to store enums as ordinal and string and when to store enum types to …