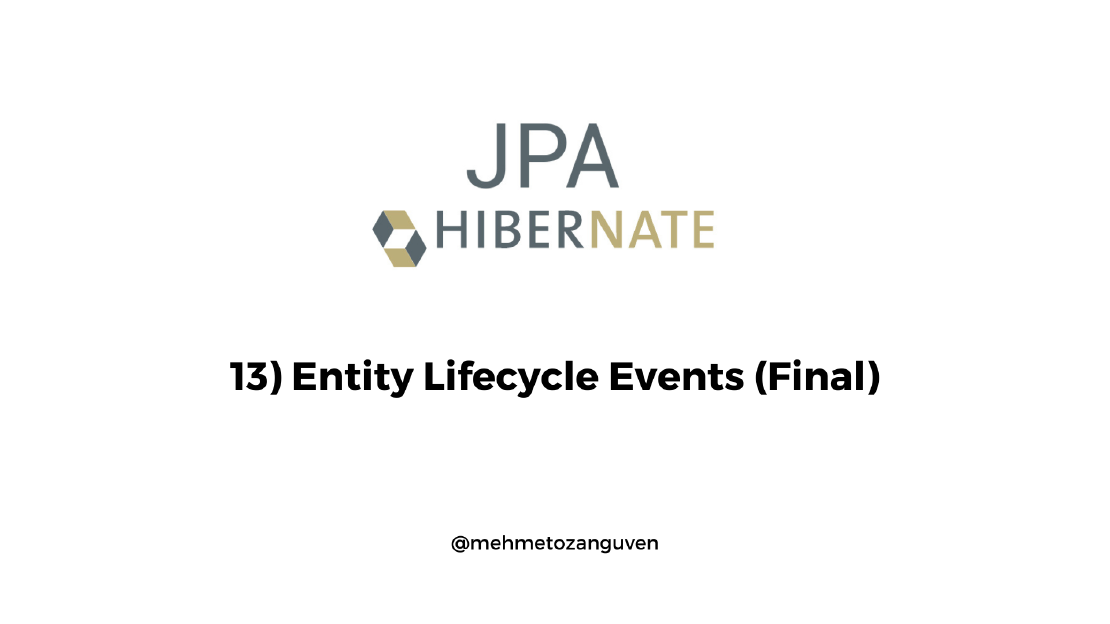
JPA Fundamentals & Hibernate - 13) Entity Lifecycle Events (Final)
In this article, we are going to learn lifecycle events in the JPA. As of the most application we will have date_created and last_modified column to …
Sometimes your entity might need store nested data structures such as Map<String, Map<String, String>
or list of list etc.. If you want to store these entities in your database, you can easily do that in hibernate 6.
Let’s say you want to map the following entities into your database (could be PostgresSQL):
@NoArgsConstructor
@Getter
@Setter
@ToString
public class UserJobDataEntity {
// ... id and other fields ...
@Column(name = "jobId", unique = true)
@NotBlank
private String jobId;
@Column(name = "resources")
private Map<String, Set<String>> setOfResources = new HashMap<>();
}
If you run the project with the setup above, you will encounter an error. Because JPA can’t map the nested structure to the database. To persist entity, you can convert the nested structure to JSON string which Hibernate will automatically convert to the proper structure when you retrieve the data from the database.
To convert the structure to the JSON string, you can use @JdbcTypeCode(SqlTypes.JSON)
annotation. Here’s the updated entity class:
@NoArgsConstructor
@Getter
@Setter
@ToString
public class UserJobDataEntity {
// ... id and other fields ...
@Column(name = "jobId", unique = true)
@NotBlank
private String jobId;
@JdbcTypeCode(SqlTypes.JSON) // Hibernate 6 will automatically handle JSON conversion
@Column(name = "resources")
private Map<String, Set<String>> setOfResources = new HashMap<>();
}
Note: If you’re using Hibernate 5, you can still convert the nested data structure to a JSON string, but it requires more steps than the annotation solution. You can search the internet for more information on how to do this.
In this article, we are going to learn lifecycle events in the JPA. As of the most application we will have date_created and last_modified column to …
In this article, we will learn what is the JPQL(The Java Persistence Query Language) and how to use it. What is the JPQL The Java Persistence query …