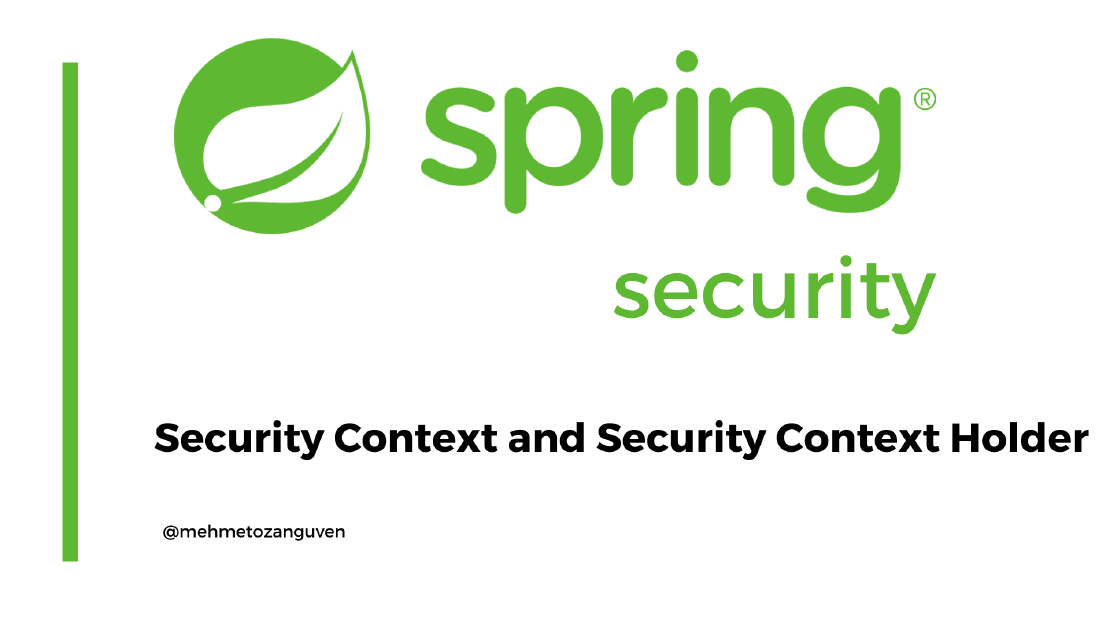
Spring Security -- 7) Security Context and Security Context Holder
In this post, let’s find out what the Security Context is I am going to use the project that I have implemented in the previous post. Here is …
Recently I have encountered this error. And I can not send any request even I have setup corsConfiguration.setAllowedOrigins("*")
. Response was only saying => Invalid CORS request
Checkout my udemy course https://www.udemy.com/course/learning-spring-security-fundamentals/ if you really want to know fundemantals of the Spring Security and more …
Solution is that if you setup your cors configuration through CorsFilter you should set the allowed methods.
Here is the example:
@Component
@EnableWebSecurity
public class SecurityConfiguration extends WebSecurityConfigurerAdapter {
@Bean
public CorsFilter corsFilter() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
CorsConfiguration corsConfiguration = new CorsConfiguration();
corsConfiguration.setAllowedHeaders(List.of("*"));
corsConfiguration.setAllowedOrigins(Arrays.asList("*"));
corsConfiguration.setAllowedMethods(Arrays.asList("*")); // add this line with appropriate methods for your case
corsConfiguration.setMaxAge(Duration.ofMinutes(10));
source.registerCorsConfiguration("/**", corsConfiguration);
return new CorsFilter(source);
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable();
http.authorizeRequests().anyRequest().permitAll();
http.cors();
}
}
In the DefaultCorsProcessor.handleInternal(...)
method:
protected boolean handleInternal(ServerHttpRequest request, ServerHttpResponse response,
CorsConfiguration config, boolean preFlightRequest) throws IOException {
// ...
HttpMethod requestMethod = getMethodToUse(request, preFlightRequest);
List<HttpMethod> allowMethods = checkMethods(config, requestMethod);
if (allowMethods == null) {
logger.debug("Reject: HTTP '" + requestMethod + "' is not allowed");
rejectRequest(response);
return false;
}
// ...
responseHeaders.setAccessControlAllowOrigin(allowOrigin);
// ...
}
protected void rejectRequest(ServerHttpResponse response) throws IOException {
response.setStatusCode(HttpStatus.FORBIDDEN);
response.getBody().write("Invalid CORS request".getBytes(StandardCharsets.UTF_8));
response.flush();
}
In this post, let’s find out what the Security Context is I am going to use the project that I have implemented in the previous post. Here is …
In this post, let’s implement two steps authentication mechanism. This will be similar to JWT authentication but instead of JWT I will use my …