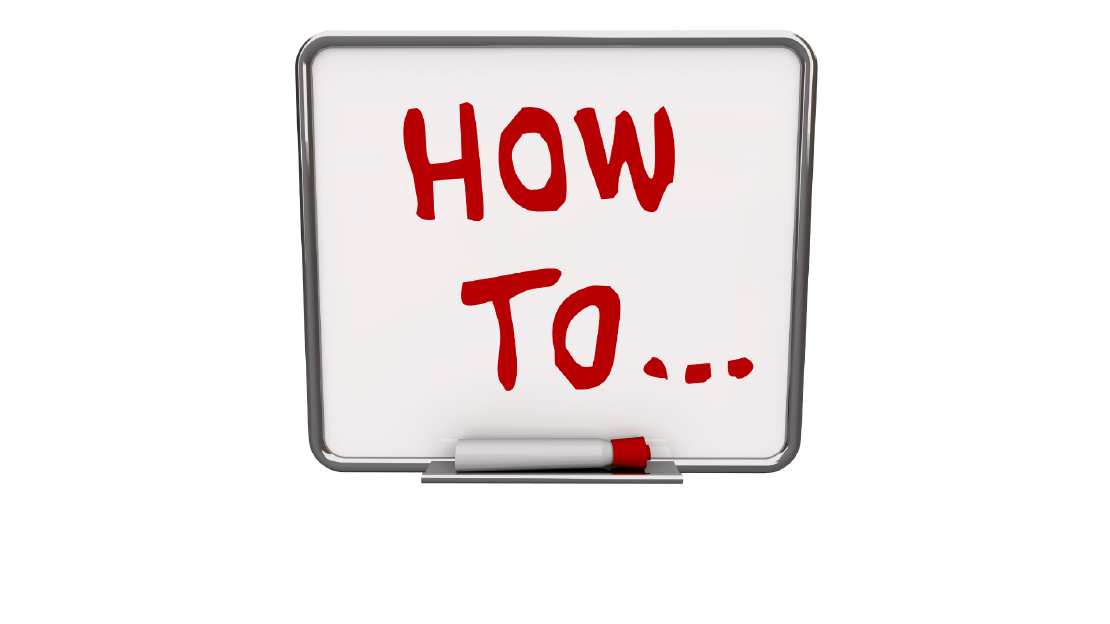
How to validate object programmatically in Spring Boot
Validating objects is crucial to ensure data integrity and prevent errors in subsequent layers of the application. While the @Valid annotation is …
Most probably you have encounter the following error, while waiting response from other services(for instance it could be payment response from the integrator):
{
"status": 415,
"error": "Unsupported Media Type",
"exception": "org.springframework.web.HttpMediaTypeNotSupportedException",
"message": "Content type 'application/x-www-form-urlencoded;charset=UTF-8' not supported"
}
And most probably your endpoint is:
public class PaymentResponse {
@PostMapping(value = ...)
public String responseFromIntegrator(@RequestBody ResponseFromIntegrator reqBody) {
// ...
}
}
The problem is that when request’s media type is application/x-www-form-urlencoded, Spring boot doesn’t understand it as a RequestBody. To solve that problem we need to say to Spring Boot “accept the application/x-www-form-urlencoded request for the endpoint”
application/x-www-form-urlencoded request?
means that the HTTP POST request body is expected to be in the form URL-encoded format. In this format, the data is sent as key-value pairs, where the keys and values are URL-encoded. For example:
name=John+Doe&age=25&email=johndoe%40example.com
By setting the consumes field into the @PostMapping
annotation right now spring boot accepts form-urlencoded request also: @PostMapping(consumes = {MediaType.APPLICATION_FORM_URLENCODED_VALUE})
By specifying
consumes = {MediaType.APPLICATION_FORM_URLENCODED_VALUE}
in the @PostMapping annotation, the method in the Spring Boot controller expects the request body to be form URL-encoded format.
But updating the annotation isn’t enough, we should also remove @RequestBody ResponseFromIntegrator reqBody
because this has no relatioship with application/x-www-form-urlencoded request.
At the end our post request should be like this:
Spring Boot automatically converts the form URL-encoded data into the params parameter, which is a
Map<String, String>
containing the key-value pairs from the request body
public class PaymentResponse {
@PostMapping( consumes = {MediaType.APPLICATION_FORM_URLENCODED_VALUE})
public String responseFromIntegrator(HttpServletRequest httpServletRequest, @RequestParam Map<String, String> params) {
String value = params.get("payment_field");
}
}
Validating objects is crucial to ensure data integrity and prevent errors in subsequent layers of the application. While the @Valid annotation is …
Jackson is a popular Java library for working with JSON. Even it is easy to use, when converting a JSON string to a generic Java class, such as …